It's sometimes useful to saturate a network with simple traffic to test your QoS measures. What you want is something that'll send a controlled amount of bandwidth. A TCP-friendly connection won't work, because TCP slows down to the available amount of bandwidth. iperf from NLANR is one such tool. However, you have to have it running on both the sender and the receiver.
I couldn't find anything quick and easy that would do it. Here's a tool that does it. You'll need Perl and Time-HiRes to use it.
#!/usr/bin/perl -w ## Mark R. Lindsey, lindsey@acm.org ## Flood a destination with fixed-sized UDP packets. use Time::HiRes qw( usleep ualarm gettimeofday tv_interval ); use IO::Socket; $number_of_args = $#ARGV + 1; if ($number_of_args < 3) { print "Usage: flood_udp.pl target_ip target_udp_port packets_per_second\n" ; die "Not enough arguments" } $target = $ARGV[0]; $target_port = $ARGV[1]; $packets_per_second = $ARGV[2]; $interpacket_delay_microseconds = 1 / $packets_per_second * 1000000; socket(BLAST, PF_INET, SOCK_DGRAM, getprotobyname("udp")); ## This assumes a 1500-byte path MTU. The idea is to make a maximum-sized packet without fragmentation. ## But if there's some fragmentation, that's not all so terrible. my $payload_size = 1472; my $ip_packet_size = $payload_size + 8; ## assuming Ethernet $msg = "u" x $payload_size; my $bytes_per_second = $ip_packet_size * $packets_per_second; my $bits_per_second = $bytes_per_second * 8; print "$bits_per_second bits/second, $bytes_per_second bytes/second\n"; print "Sending to $target at UDP port $target_port\n"; ## Send the UDP packet $ipaddr = inet_aton($target); $sendto = sockaddr_in($target_port,$ipaddr); while (1) { send(BLAST, $msg, 0, $sendto) ; usleep ($interpacket_delay_microseconds); }
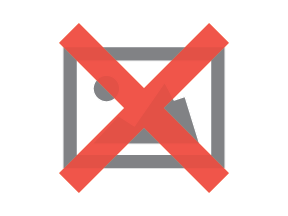